Tailwind CSS setup with ReactJs
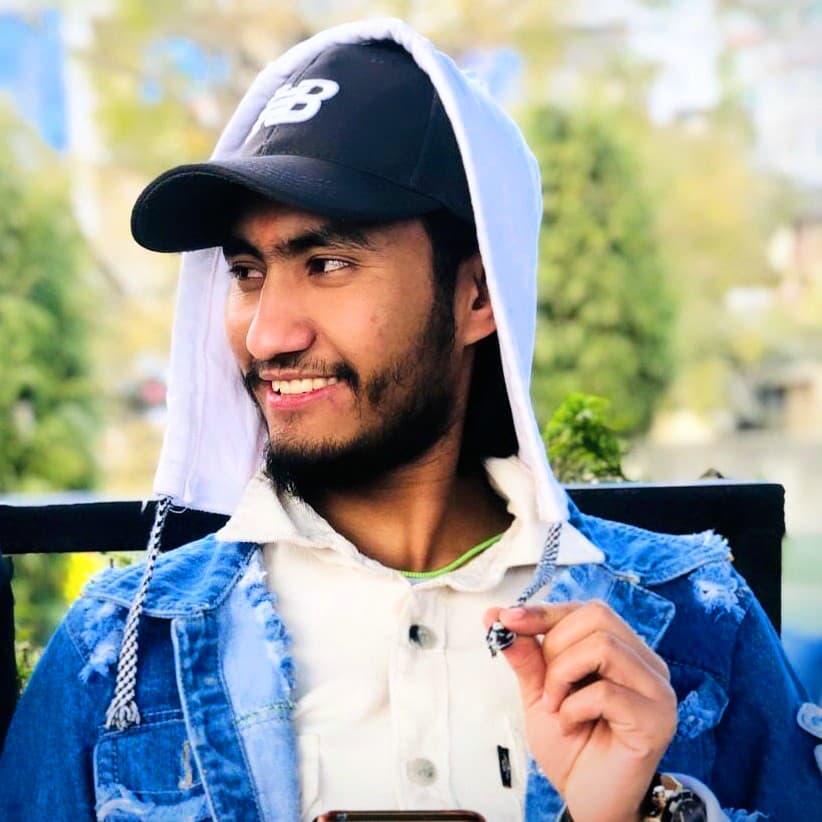
Gokarna Adhikari
Mar 22, 2024•3 min read
Introduction
In the world of web development, efficiency and flexibility are key. In this guide, we'll explore how to set up a robust development environment using Tailwind CSS, React.js, and Vite.js. Let's start by understanding each of these technologies:
1. Definition of Tailwind CSS, React.js, and Vite.js:
-
Tailwind CSS: Tailwind CSS is a utility-first CSS framework that provides pre-designed utility classes to rapidly build custom designs. It allows developers to create modern UIs without writing custom CSS, promoting a modular approach to styling.
-
React.js: React.js is a JavaScript library for building user interfaces. It employs a component-based architecture, where UIs are broken down into reusable components, promoting a declarative and efficient programming paradigm.
-
Vite.js: Vite.js is a modern build tool for web development. Leveraging native ES module imports, Vite.js offers blazing-fast development and build times by serving the source code directly to the browser during development without bundling.
2. Advantages and Disadvantages:
Advantages:
-
Rapid Development: Tailwind CSS speeds up development by providing pre-designed utility classes.
-
Component Reusability: React.js promotes component-based development, enhancing code reusability and maintainability.
-
Fast Development Server: Vite.js offers near-instantaneous hot module replacement (HMR) and optimized development builds, enhancing the developer experience.
Disadvantages:
-
Learning Curve: Tailwind CSS may have a learning curve for developers accustomed to traditional CSS.
-
Build Configuration: Configuring Tailwind CSS and Vite.js may require additional setup compared to conventional tools.
-
Performance Overhead: Tailwind CSS generates large CSS files, which can affect performance if not optimized properly.
3. Installation Guide:
Step 1: Initialize a New React.js Project:
npx create-react-app my-tailwind-app
cd my-tailwind-app
Step 2: Install Tailwind CSS and its Dependencies:
npm install tailwindcss postcss autoprefixer
Step 3: Configure Tailwind CSS:
Create a tailwind.config.js
file at the root of your project:
/** @type {import('tailwindcss').Config} */
export default {
content: [
"./index.html",
"./src/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Step 4: Configure PostCSS:
Create a postcss.config.js
file at the root of your project: (Note: it automatically Create on your project, You have to check that)
// postcss.config.js
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {},
},
};
Step 5: Import Tailwind CSS into your Stylesheets:
Create a styles.css
file in the src
directory and import Tailwind CSS:
* src/styles.css */
@import 'tailwindcss/base';
@import 'tailwindcss/components';
@import 'tailwindcss/utilities';
Step 6: Update React.js Entry Point:
Edit the index.css
file in the src
directory to import the styles.css
file:
// src/index.css
import './styles.css';
Step 7: Start the Development Server:
npm start
4. Creating a Simple Page:
Now that we have our environment set up, let's create a simple page using React.js and Tailwind CSS:
Step 1: Edit the App.js
file:
Replace the contents of App.js
with the following code:
// src/App.js
import React from 'react';
function App() {
return (
<div className="bg-gray-100 h-screen flex items-center justify-center">
<h1 className="text-4xl font-bold text-blue-500">Hello, Tailwind CSS!</h1>
</div>
);
}
export default App;
Step 2: Start the Development Server:
npm start
Visit http://localhost:3000
in your browser to see the simple page we just created with Tailwind CSS and React.js.
Please Visit official website of Tailwind CSS: https://tailwindcss.com/docs/installation/framework-guides
Tags